Understanding Middleware in Laravel: A Comprehensive Guide
- seoiphtechnologies
- Jul 29, 2024
- 2 min read
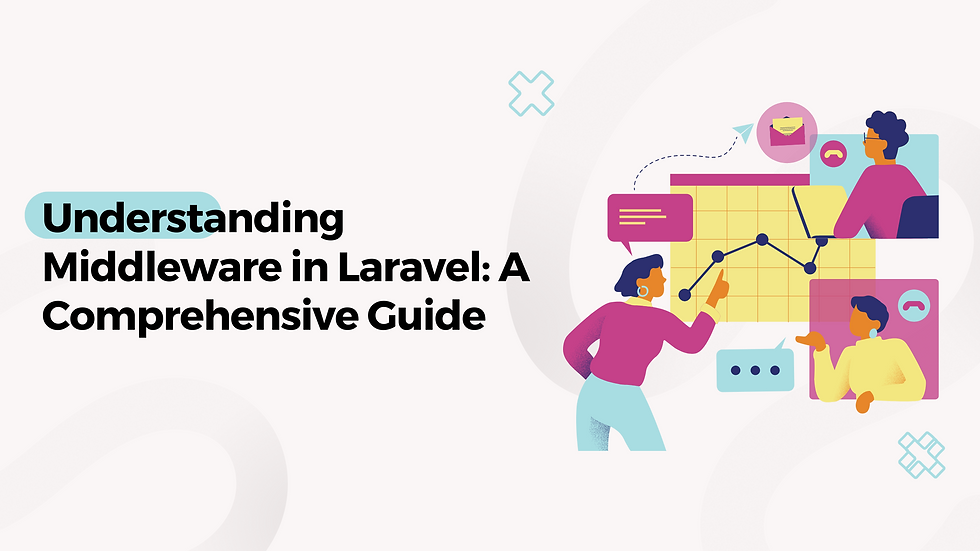
Middleware in Laravel is a powerful tool that allows you to filter HTTP requests entering your application. It provides a convenient mechanism for inspecting and filtering the incoming HTTP requests. This blog will help you understand the concept of middleware in Laravel and how to utilize it effectively.
What is Middleware?
Middleware acts as a bridge between a request and a response. It sits between the client and the server, processing the incoming request before it reaches the controller and the outgoing response before it reaches the client. Middleware can perform various tasks such as authentication, logging, and data manipulation.
Types of Middleware
Laravel provides different types of middleware:
Global Middleware: Runs on every HTTP request to the application.
Route Middleware: Assigned to specific routes to control their access.
Group Middleware: Assigned to a group of routes, enabling you to apply middleware to multiple routes at once.
Creating Middleware
To create middleware, use the Artisan command:
bash
Copy code
php artisan make:middleware CheckAge
This command will generate a new middleware class in the app/Http/Middleware directory.
Example Middleware
Here's an example of a simple middleware that checks the age of the user:
php
<?php namespace App\Http\Middleware; use Closure; use Illuminate\Http\Request; class CheckAge { /** Handle an incoming request. @param \Illuminate\Http\Request $request @param \Closure $next @return mixed / public function handle(Request $request, Closure $next) { if ($request->age <= 18) { return redirect('home'); } return $next($request); } }
In this example, the middleware checks if the user's age is less than or equal to 18 and redirects them to the home page if it is.
Registering Middleware
Once you have created your middleware, you need to register it. There are two ways to register middleware in Laravel:
Global Middleware
To register global middleware, add it to the $middleware array in app/Http/Kernel.php:
php
Copy code
protected $middleware = [ // Other middleware \App\Http\Middleware\CheckAge::class, ];
Route Middleware
To register route middleware, add it to the $routeMiddleware array in app/Http/Kernel.php:
php
protected $routeMiddleware = [ // Other middleware 'checkage' => \App\Http\Middleware\CheckAge::class, ];
Then, you can apply this middleware to specific routes in your routes/web.php file:
php
Route::get('profile', function () { // Only accessible if age is greater than 18 })->middleware('checkage');
Middleware Parameters
Middleware can also receive additional parameters. For example, you can modify the CheckAge middleware to accept a minimum age parameter:
php
public function handle(Request $request, Closure $next, $minAge) { if ($request->age <= $minAge) { return redirect('home'); } return $next($request); }
Then, you can pass the parameter when defining the route:
php
Route::get('profile', function () { // Only accessible if age is greater than 18 })->middleware('checkage:18');
Conclusion
Middleware is a fundamental part of Laravel's HTTP kernel, providing a convenient way to filter and manipulate HTTP requests. By understanding and utilizing middleware effectively, you can enhance the security, performance, and functionality of your Laravel applications.
If you need expert assistance in building and maintaining Laravel applications, consider Hire Dedicated Laravel Developers USA. Their expertise can ensure that your applications are robust, scalable, and secure.
Looking to elevate your Laravel projects? Hire Dedicated Laravel Developers USA today and see the difference they can make in your development process.
댓글