How to Optimize Performance in Laravel Applications
- seoiphtechnologies
- Jun 5, 2024
- 2 min read
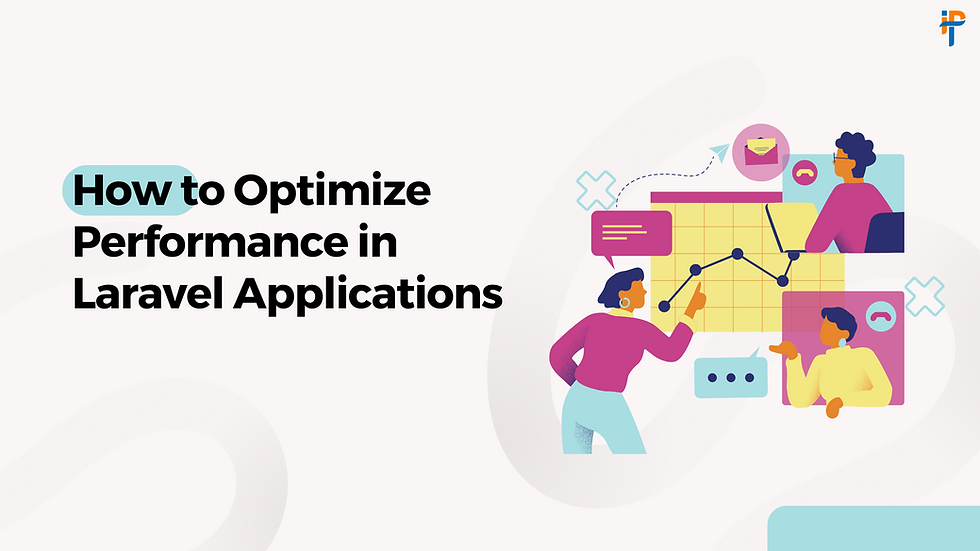
Optimizing the performance of Laravel applications is crucial for providing a seamless user experience and ensuring that your web solutions are efficient and scalable. Whether you are a Laravel development company or looking to hire Laravel developers, these tips will help you enhance the performance of your Laravel applications.
1. Use the Latest Version of Laravel
Ensure your Laravel application is running on the latest version. Each release comes with performance improvements and new features that can help optimize your application. Staying updated also ensures better security and support.
2. Optimize Composer Autoload
Using Composer's optimized autoload can improve performance by reducing the load time of your classes. Run the following command:
bash
Copy code
composer dump-autoload -o
This command generates a class map for faster autoloading.
3. Cache Configurations and Routes
Caching configurations and routes can significantly reduce the application's response time. Use the following Artisan commands to cache your configurations and routes:
bash
Copy code
php artisan config:cache php artisan route:cache
These commands compile all configurations and routes into a single file, speeding up the application.
4. Use Eager Loading to Optimize Queries
Eager loading can prevent the N+1 query problem by loading all necessary relationships upfront. Instead of lazy loading:
php
Copy code
$users = User::with('posts')->get();
Using eager loading reduces the number of queries and improves performance.
5. Minimize Middleware Usage
Only apply middleware where necessary to avoid unnecessary processing. Group your routes and apply middleware only to those groups that require it. This ensures that middleware checks are not performed on every request.
6. Optimize Database Queries
Efficient database queries are essential for performance. Use indexes on frequently queried columns, avoid using SELECT *, and leverage Laravel’s query builder for more complex queries. Additionally, consider using database caching solutions like Redis or Memcached.
7. Utilize Laravel's Built-in Cache
Laravel provides a robust caching system that can be used to store frequently accessed data. Use cache drivers like Redis or Memcached for better performance. Here’s how you can cache a query result:
php
Copy code
$users = Cache::remember('users', 60, function () { return DB::table('users')->get(); });
8. Implement Queues for Time-Consuming Tasks
Offload time-consuming tasks like sending emails, generating reports, or processing uploads to background queues. This will improve the response time for your users. Laravel's queue system makes this process straightforward:
php
Copy code
dispatch(new SendEmailJob($user));
9. Use Content Delivery Networks (CDNs)
Serving static assets like images, CSS, and JavaScript files through a CDN can significantly improve load times. CDNs reduce the load on your server and deliver content faster by using distributed servers.
10. Monitor and Profile Your Application
Regularly monitoring and profiling your application can help identify bottlenecks. Use tools like Laravel Telescope, Blackfire, or Xdebug to analyze performance and find areas for improvement.
Conclusion
Optimizing Laravel applications involves a combination of best practices and strategic enhancements. By implementing these tips, you can ensure that your application runs smoothly and efficiently. If you're looking for expert help, consider partnering with a Laravel development company that offers laravel development services or hire Laravel developers with proven expertise in performance optimization.
By focusing on these optimization techniques, your Laravel applications will not only perform better but also provide an enhanced user experience, contributing to the success of your business.
Opmerkingen