Building High-Performance Apps with Flutter: Best Practices
- seoiphtechnologies
- Sep 6, 2024
- 4 min read
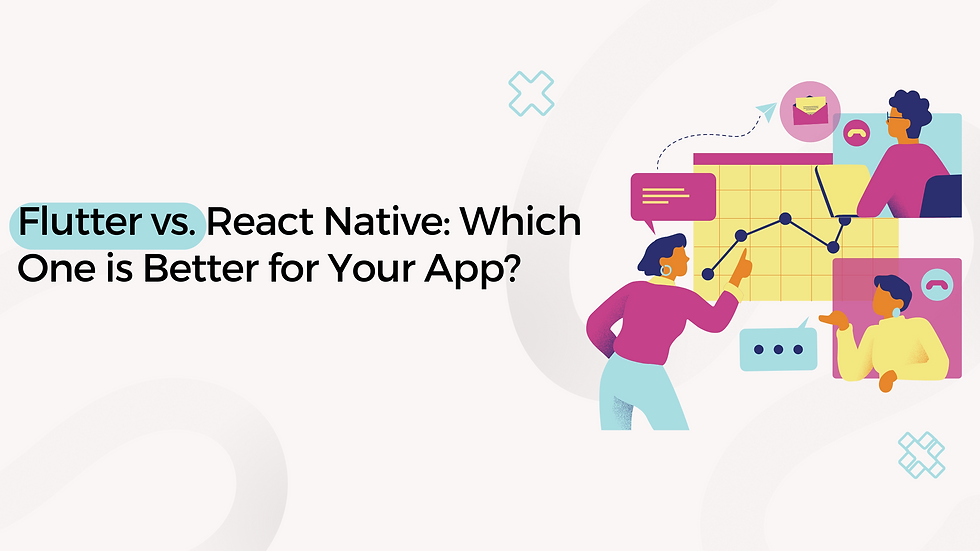
In the highly competitive app development landscape, performance plays a pivotal role in the success of any application. Flutter, Google’s open-source UI framework, allows developers to build natively compiled applications for mobile, web, and desktop from a single codebase. One of the most compelling aspects of Flutter is its ability to deliver high-performance apps. However, building apps with peak performance requires more than just Flutter’s powerful framework. It demands implementing best practices to ensure a smooth, responsive, and efficient app experience.
Here are some of the best practices for building high-performance apps with Flutter.
1. Optimize Widget Trees
In Flutter, everything is a widget, and the structure of your widget tree has a significant impact on the performance of your app. Overly complex or deep widget trees can slow down rendering and negatively affect the overall user experience. Here are some tips to optimize your widget trees:
Minimize Rebuilds: Avoid rebuilding the entire widget tree when only part of it changes. You can achieve this by splitting your widget into smaller, more manageable components and using Flutter's const constructor whenever possible.
Use Stateless Widgets Wisely: Whenever possible, use StatelessWidget instead of StatefulWidget if your widget doesn’t need to manage any state. Stateless widgets are more efficient because they don’t need to trigger any rebuilds.
Avoid Unnecessary Parent Widgets: Try to reduce unnecessary parent widgets, as each widget adds to the complexity of the widget tree. For example, avoid using multiple Padding or Container widgets unnecessarily.
2. Use Asynchronous Programming for Heavy Tasks
Long-running tasks such as fetching data from the internet, reading files, or performing CPU-intensive operations can cause performance bottlenecks if they block the main UI thread. Dart, the language used by Flutter, provides a powerful asynchronous programming model using Future and async/await. Here are some best practices:
Async/Await: Use async functions and await for long-running tasks to prevent blocking the UI.
Isolate for Heavy Computations: For CPU-bound tasks, consider using Isolate. Flutter apps run in a single-threaded environment, and isolates allow you to run heavy tasks on separate threads, preventing any lag in the UI.
3. Reduce Overdraw and Excessive Repaints
Overdraw occurs when the same pixel is rendered multiple times in a single frame. This can lead to inefficient rendering and degrade app performance. To avoid overdraw:
Use Opacity Widgets Sparingly: Excessive use of Opacity widgets can cause multiple layers to be painted unnecessarily. Instead, use a widget’s color or alpha properties to achieve similar effects without layering.
Cull Offscreen Widgets: Widgets that are off-screen or not currently visible can still impact performance. Use ListView.builder or similar widgets to lazily load content and ensure you’re not building or painting widgets that users can't see.
Avoid Unnecessary Repaints: Repainting unnecessary parts of your UI is one of the most common performance bottlenecks. Use RepaintBoundary to isolate the parts of the UI that need to be repainted.
4. Optimize Network Requests
Flutter apps often rely on network requests for fetching data, but inefficient network handling can lead to sluggish performance. Best practices include:
Caching Data: Implement proper caching mechanisms to reduce network calls. This will improve performance, especially when fetching large amounts of data.
Batching Network Requests: Minimize the number of requests by batching them when possible. This reduces latency and improves responsiveness.
Use Efficient APIs: Ensure that you’re using efficient APIs for data fetching and avoid over-fetching. This will not only improve performance but also reduce the load on your backend.
5. Leverage Flutter’s Performance Tools
Flutter offers a variety of tools to help diagnose and fix performance issues. Some essential tools include:
DevTools: Flutter’s DevTools provide insights into app performance, helping you identify and resolve performance bottlenecks such as excessive rebuilds or memory leaks.
Flutter Inspector: This tool helps visualize and debug the widget tree and understand how your app renders UI components.
Profile Mode: Always run your app in profile mode to get an accurate measurement of how it performs in a real-world environment.
6. Image and Asset Optimization
Large images and unoptimized assets can slow down the performance of your app, especially on lower-end devices. Here are some tips to optimize assets:
Resize Images: Always resize images to the appropriate dimensions before including them in your app. Large images can consume memory unnecessarily and slow down performance.
Use WebP Format: For images, consider using the WebP format, which offers better compression than PNG or JPEG without losing image quality.
Lazy Loading: For image-heavy apps, use lazy loading techniques to load images only when they are needed, reducing memory usage and improving performance.
7. Minimize Package and Plugin Usage
While Flutter’s extensive ecosystem of packages and plugins is a great asset, using too many can slow down your app. Be selective about the plugins you incorporate, and avoid those that add unnecessary overhead.
Conclusion
Building high-performance apps with Flutter is not just about using the framework—it’s about implementing best practices that optimize your app’s responsiveness and efficiency. By optimizing widget trees, leveraging asynchronous programming, reducing overdraw, optimizing network requests, and using Flutter’s built-in performance tools, you can create apps that offer a seamless user experience, even on resource-constrained devices.
These best practices are essential for anyone looking to build scalable, fast, and efficient apps using Flutter. Whether you’re a startup or an established enterprise, focusing on performance will ensure your Flutter app stands out in today’s competitive market.
For more information on Flutter App Development and how to maximize its potential, reach out to expert developers or communities dedicated to Flutter.
Коментари